Basic CakePHP templating skills
Posted on 11/10/06 by Felix Geisendörfer
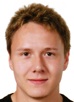
One of the things I don't see getting to much coverage is how to create good templates when working with CakePHP. Since those are written in plain PHP, this does not apply to CakePHP only. So I'm sure many people have already developed their own style that they are comfortable with and I don't ask for them to change it. However, maybe some people new to the framework / language can benifit by taking a look at the one I'm using.
PHP tags
The first thing I recommend is using the fully qualified syntax (<?php ?>) for php tags instead of the short one (<? ?>). Why? Well that's what the CakePHP coding standards recommend you to use, and it makes those statments stand out a little more in your html code. But the main reason still is that the short tags syntax can be turned off via php.ini, so using them makes your app less portable and could cause raw code output incidents.
Conditions
While you are used to use to wrap conditional commands in curly braces in your normal PHP code, they are not quite as practical in templates. Instead you should make use of this syntax which makes your code easier to read & write:
<?php else: ?>
<p>The name of the User you are looking for is: <?php echo $user['User']['name']; ?></p>
The advantage of this is that you don't have to use curly braces to wrap your command blocks, but instead simply use if, else and endif to do so.
Loops
When working with CakePHP the foreach loop is your best friend. A lot of things are done via associative arrays and even plain numerical arrays are quite comfortable to process this way. The syntax I recommend is the same one as for if statements:
<?php echo $event['Event']['html'] ?>
The linebreak issue
Now when using loops (or several inline statements) and manually viewing your html output, you'll often notice that line breaks are apparantly swallowed, and everything is on one line. Well this is not CakePHP's fault, but rather the default PHP behavior. Whenever an inline statement ends with ?> followed by a line break, this line break will not appear in the output. But don't you worry, there is a simple fix for this: Simply add a space in between ?> and the line break and voila, the output turns out as expected.
Avoid multi-line statements
One thing I found usefull when writing templates was to adobt an "avoid multi-line commands" policy. This means that you should do one command per inline statement, and not several ones at once. On occassions I break this rule, but most of the time it serves as a very good indicator for showing you that you using Controller logic in your views, or you should start writing a Helper for something. The worst thing you can do, is define functions inside your View. Not only should those be moved to helpers, but when this view get's rendered twice in one request you'll get a nasty php error, telling you you can't define the function twice ...
Creating Zebra striped table rows
This is a little specific, but I've seen some really horrible approaches for assigning varying css classes to every row in the past, so this is how I would recommend you to do it:
<td><?php echo $event['Event']['title']; ?></td>
... More columns go here ...
</tr>
Since only every 2nd number in a consecetuive series of integer values can be divided by 2 without leaving a remainder (even numbers), this can be used to constantly switch the css class between 'row-a' and 'row-b'.
Alright, I hope this little tutorial will help some new folks, and maybe some others as well.
--Felix Geisendörfer aka the_undefined
You can skip to the end and add a comment.
Daniel, yeah, I often do that. But for short examples like the ones above I find myself more happy to skip them, but again - that's just a personal preference.
Aaah, didn't know the line break thingy, ty Felix!
just wanted to suggest a change in your zebra-striped table:
better use
($num % 2 == 0)
this way it's more clear, that this is an if-statement
The way you have it, it's working the other way around: The statement is true, when the modulo equals the int 1 (1,3,5,7, ...), which is then interpreted as true in php. Might be confusing.
And thx about the linebreak thing, did not know that either :-)
($num % 2 == 0) ---> now that's ugly code, especially for a types-less language like php (yes yes not really types-less but you know what i mean)
anybody who is past the novice level of php programming will understand the code without the "== 0"
just my 2 cents ;-)
Why do that at all, there is a function in cake that already takes care of that:
https://trac.cakephp.org/browser/branches/1.1.x.x/cake/libs/view/helpers/html.php#L480
Cheers,
AD7six
Ad7six: Err don't get me wrong, but when trying to display a table Helpers are kind of getting into my way. I only use them for repetitive tasks that have small interface needs, for everything else I prefer elements.
The linebreak is definitly a killer hint :-)
i used to add ."\n" to every echo, well not anymore!
I think stripped rows made by a client side framework its better to clean your code. With Jquery, for example, this could be done by one line and inserted in a external javascript. But whatever, good code pratices, it helps me a lot...
Alexandre Magno: Not everybody has JS enabled. If you need your site to look & feel good for all visitors using JS for the striped rows is no option. I do agree however that there are situations where you can use JS for this.
Using the modulo for table is awesome. It almost seems obvious now that you've pointed it out, nice and easy. And I agree for php it's prettier without the '== 0'. Been also checking out your ant article. I did some Java for none web apps and always wanted to get into. Ironic that I'm only getting into now that I've not touched Java for almost a year! Thanks for all the great info, you made my cake baking that much more enjoyable!
The line: < ?php echo ($num % 2) ? 'row-a': 'row-b'; ?>">>
Could be :
< ?
if($num ++ >0){ $class = 'oddRow'}else{ $class = 'evenRow'};>
//the you can use the class anywhere
?>
thanks, it's very useful for me :)
Doesn't #2 "Conditions" go against the CakePHP coding standard? https://trac.cakephp.org/wiki/Developement/CodingStandards#Controlstructures
This post is too old. We do not allow comments here anymore in order to fight spam. If you have real feedback or questions for the post, please contact us.
Just a detail, but I would use indentations for conditions and loops.